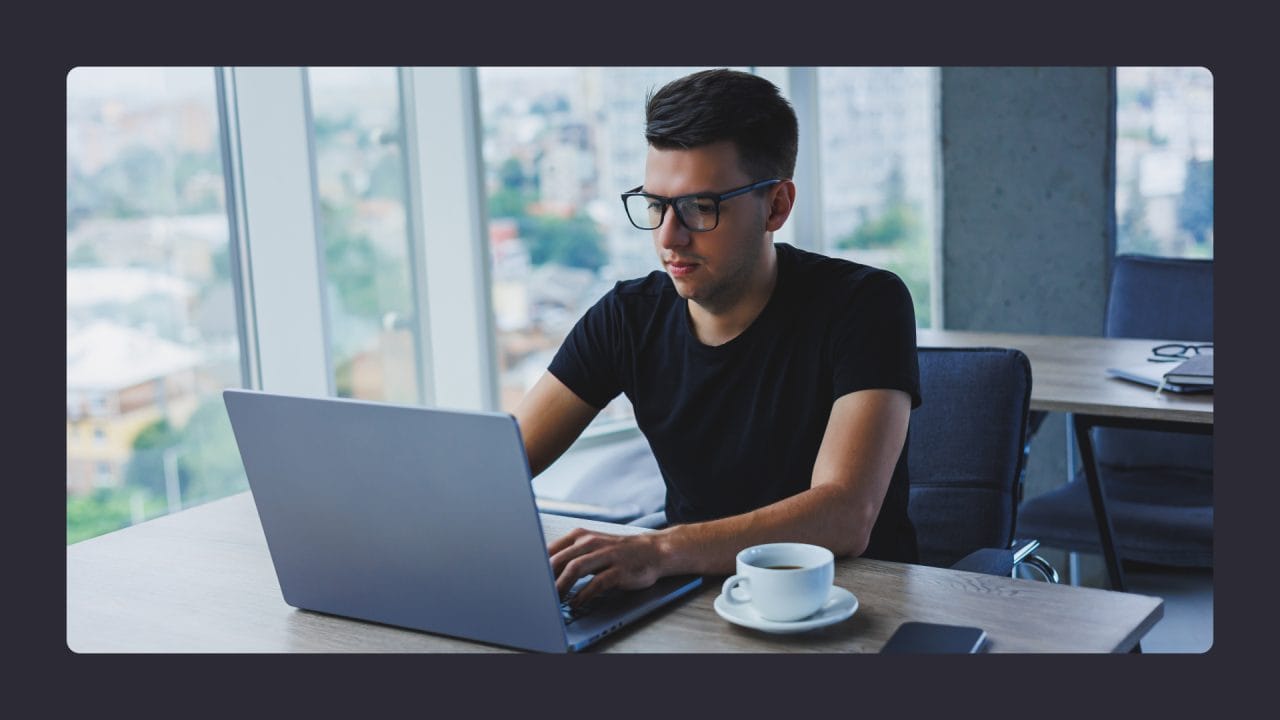
JavaScript powers dynamic and interactive web experiences, enabling developers to create engaging websites that respond to user actions. It allows for real-time updates, form validation, and seamless content changes without page reload, making it essential for building interactive websites where users can directly interact by clicking buttons or filling out forms. This versatile language works alongside HTML and CSS to enhance user interfaces and improve overall functionality.
Web developers use JavaScript to manipulate the Document Object Model (DOM), which represents the structure of web pages. This manipulation allows for adding, removing, or modifying elements on the fly. JavaScript also handles events triggered by user interactions such as clicks, keyboard input, and mouse movements, making websites more responsive and user-friendly.
Modern JavaScript frameworks and libraries extend its capabilities, offering pre-built components and tools for creating complex web applications. These resources simplify the development process and enable the creation of sophisticated interactive features with less code.
Key Takeaways
- JavaScript is essential for creating dynamic and interactive websites that engage users. It enables real-time content updates, form validation, and seamless changes without requiring page reloads. This versatile language complements HTML and CSS to enhance user interfaces and functionality.
- JavaScript allows developers to manipulate the Document Object Model (DOM), which represents the structure of web pages. This manipulation enables the dynamic addition, removal, or modification of elements. JavaScript also handles user interactions such as clicks, keyboard input, and mouse movements, making websites more responsive and user-friendly.
- Developers can leverage modern frameworks and libraries to extend JavaScript’s capabilities and streamline the development process. These tools provide pre-built components and resources that simplify creating complex web applications. By utilising these frameworks, developers can focus on building sophisticated interactive features with less code.
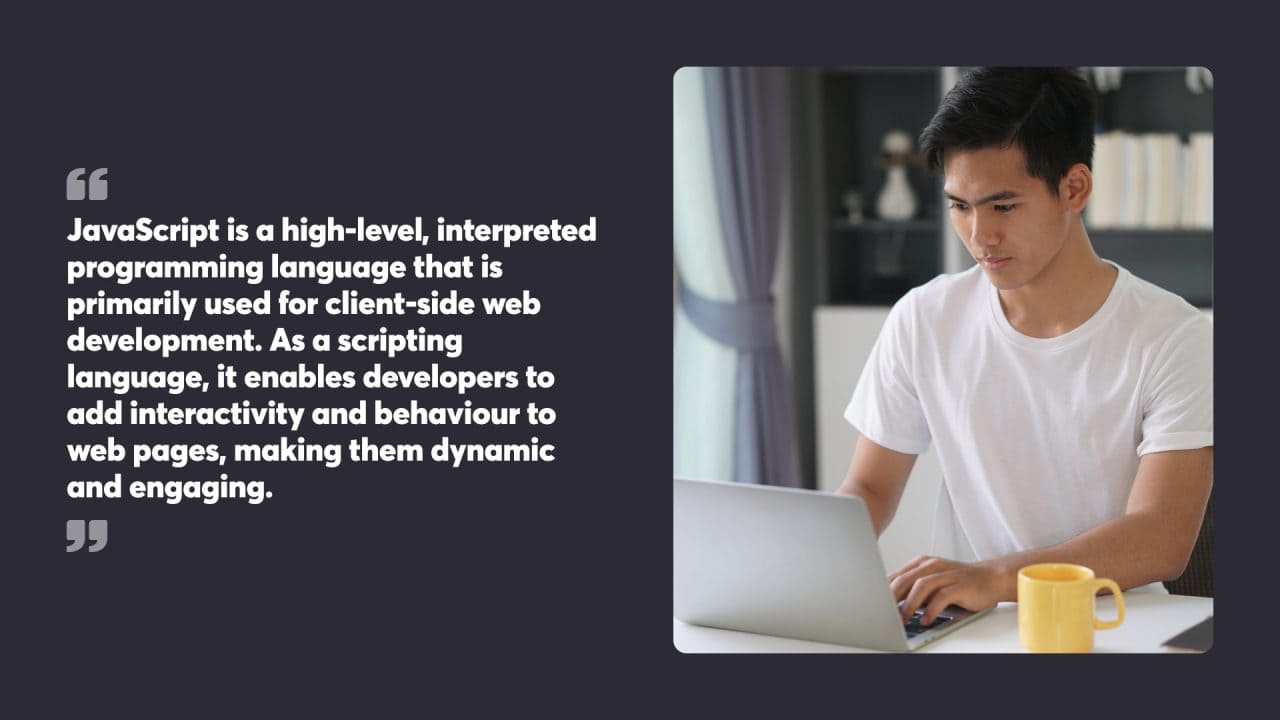
Table of Contents
What is JavaScript?
JavaScript is a high-level, interpreted programming language primarily used for client-side web development. As a scripting language, it enables developers to add interactivity and behaviour to web pages, making them dynamic and engaging. Often abbreviated as JS, JavaScript is a key component of web development, allowing developers to create interactive and dynamic web pages.
With JavaScript, developers can control the behaviour of the page’s elements, respond to user inputs, and update content dynamically. This powerful tool is indispensable for web development, enabling the creation of interactive elements such as sliders, forms, animations, and even games. By leveraging JavaScript, websites can come to life, providing a richer and more engaging user experience.
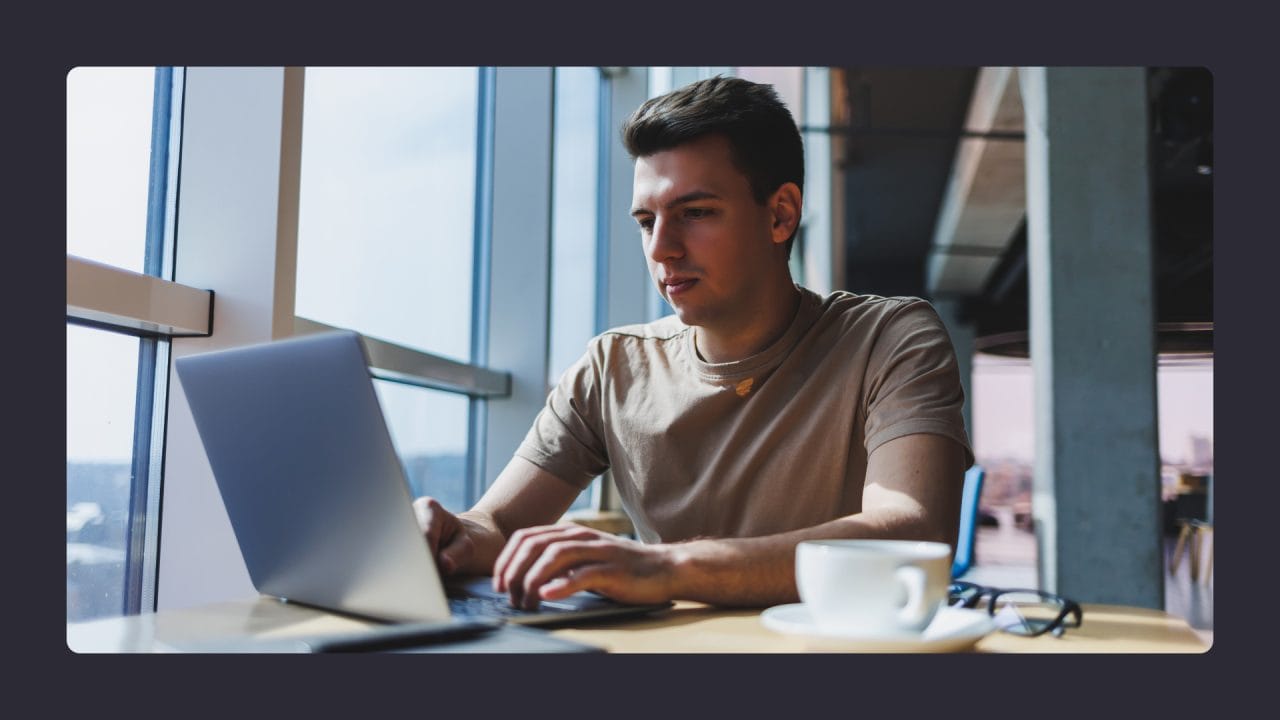
Understanding JavaScript Basics
JavaScript is a versatile programming language essential for creating interactive websites and web pages. It offers a range of tools and features for manipulating website elements and handling user interactions.
Syntax and Data Types
JavaScript syntax consists of statements that define how the code should behave. Variables are declared using keywords like var
, let
, or const
. The language supports several data types: numbers, strings, booleans, arrays, and objects.
Numbers can be integers or floating-point values. Strings are text enclosed in single or double quotes. Booleans represent true or false values. Arrays store collections of data, while objects hold key-value pairs.
JavaScript uses a camelCase naming convention for variables and functions. Statements typically end with a semicolon, though it’s not always required.
Control Structures
Control structures in JavaScript guide the flow of code execution. Conditional statements like if
, else if
, and else
allow for decision-making based on specific conditions.
Loops such as for
and while
enable code repetition. The for
loop is often used when the number of iterations is known, while while
loops continue until a condition is met.
Switch statements provide an alternative to multiple if
statements when comparing a value against several options. They can improve code readability in certain scenarios.
Functions in JavaScript encapsulate reusable code blocks. They can accept parameters and return values, making them flexible for various tasks.
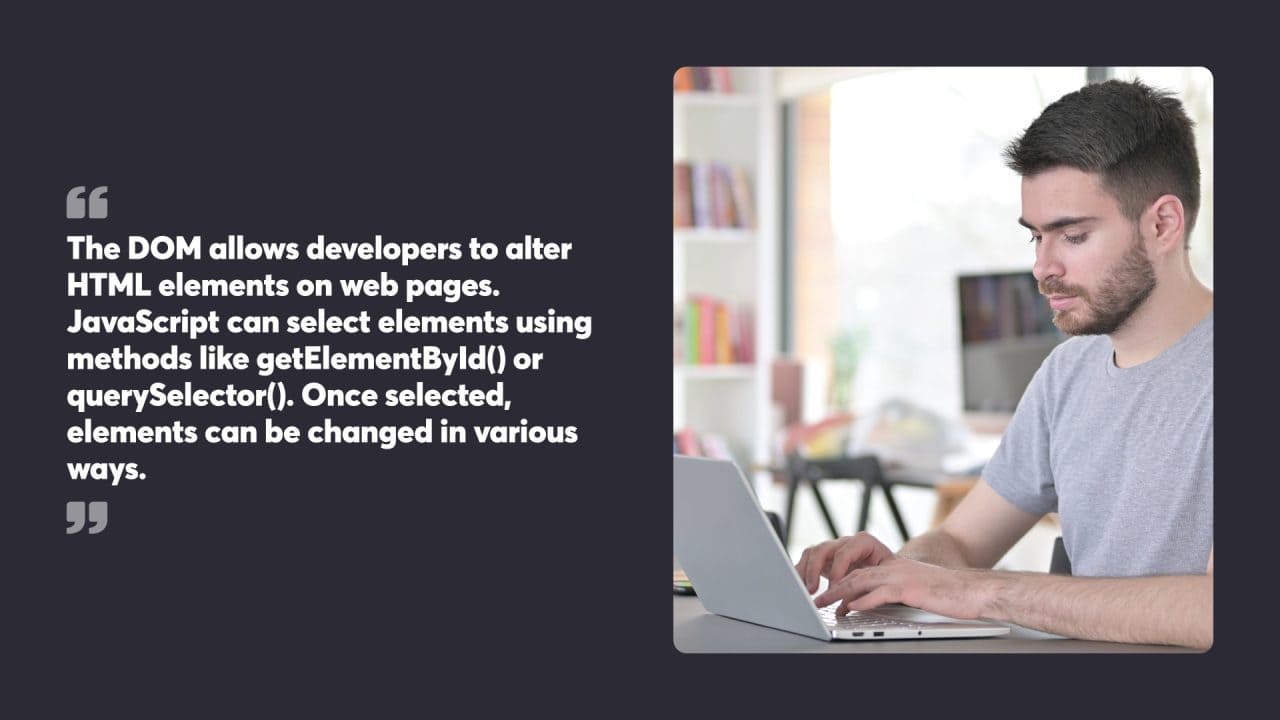
The Document Object Model (DOM)
The Document Object Model forms the backbone of interactive web pages. It provides a structured representation of HTML documents and enables JavaScript to access and modify web content dynamically.
Manipulating HTML Elements
The DOM allows developers to alter HTML elements on web pages. JavaScript can select elements using methods like getElementById()
or querySelector()
. Once selected, elements can be changed in various ways:
- Modifying content: The innerHTML property lets developers update text or HTML within an element.
- Changing attributes:
setAttribute()
andremoveAttribute()
functions adjust element properties. - Styling alterations: The style property enables direct CSS changes to elements.
DOM manipulation extends beyond single elements. Developers can create new elements with createElement()
, remove existing ones via removeChild()
, or move them around the document using appendChild()
.
Handling Events
Event handling is key to creating responsive web applications. The DOM provides an event system that allows JavaScript to react to user actions:
- Event listeners: Functions like
addEventListener()
attach code to specific events on elements. - Common events include
click
,mouseover
, andkeydown
. - Event objects contain details about the triggered event, such as mouse coordinates or pressed keys.
Developers can use event delegation to manage events efficiently. By attaching a single listener to a parent element, it can handle events for multiple child elements. This approach improves performance and simplifies code maintenance.
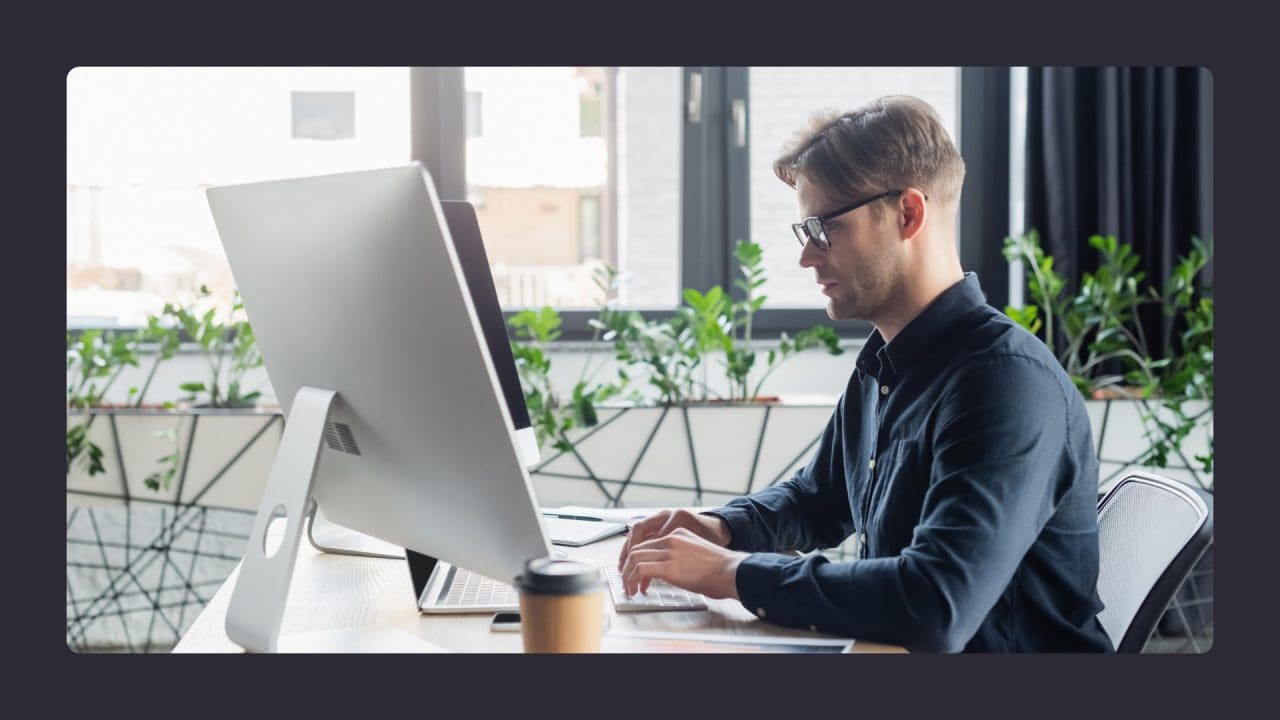
JavaScript in the Web Browser
JavaScript is crucial for creating interactive web pages. By manipulating the Document Object Model and responding to user actions, developers can enhance the user experience and build dynamic web applications.
Interacting with the User Interface
JavaScript enables developers to modify web page content and structure dynamically. DOM manipulation allows elements to be added, removed, or altered in real time. For instance, JavaScript can change text content, update styles, or create new HTML elements on the fly.
Event listeners are a key feature for interactivity. They allow JavaScript to respond to user actions like clicks, keypresses, or mouse movements. This functionality is essential for creating responsive interfaces that react to user input.
AJAX (Asynchronous JavaScript and XML) is another powerful tool. It allows web pages to update content without reloading the entire page, providing a smoother user experience. This technology is widely used in modern web applications for form submissions and data retrieval tasks.
Managing Browser Window and User Actions
JavaScript provides methods to control the browser window and handle various user actions. The Window object, representing the browser window, offers properties and methods to manage aspects like window size, scrolling, and navigation.
Developers can use JavaScript to open new windows or tabs, resize existing ones, and control the browser’s history. This capability helps create pop-ups, adjust layouts based on window size, or implement custom navigation controls.
User actions such as form submissions can be intercepted and processed using JavaScript. This allows for client-side form validation, improving user experience by providing immediate feedback without server requests.
JavaScript can also access and modify browser cookies, enabling developers to store small amounts of data on the client side. This feature is useful for remembering user preferences or maintaining session information across page reloads.
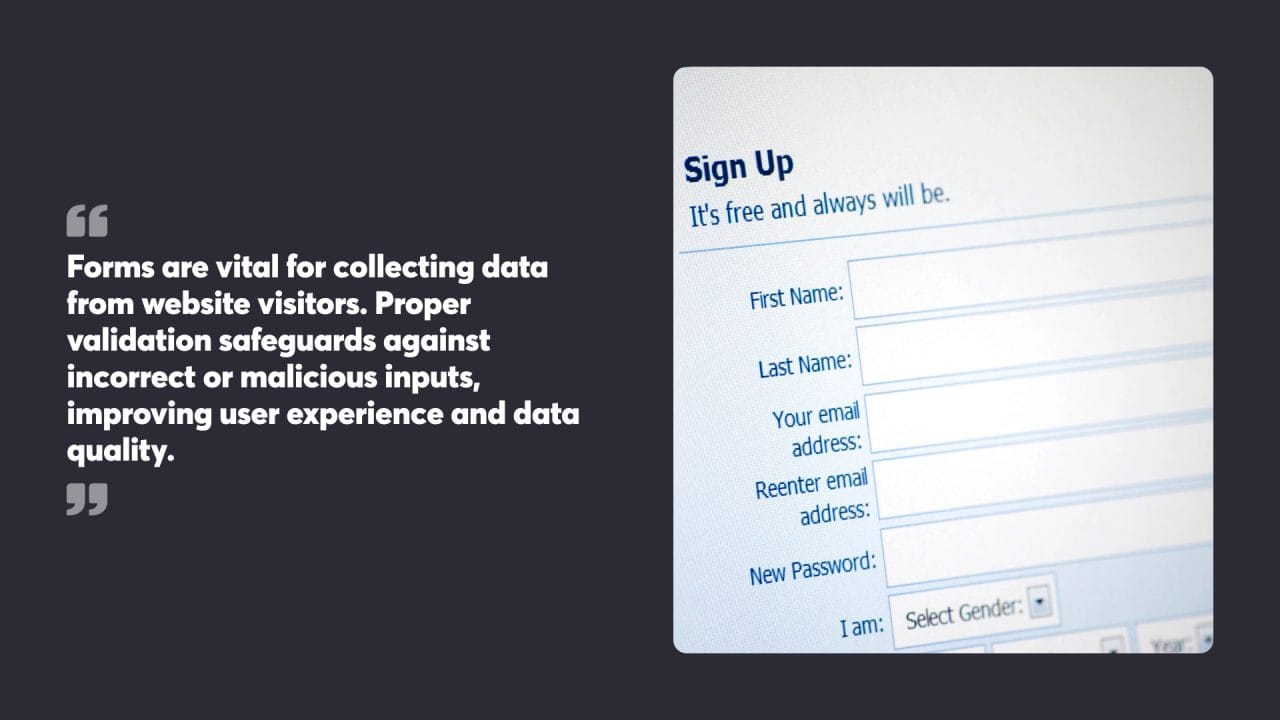
Forms and User Input Validation
Forms are vital for collecting data from website visitors. Proper validation safeguards against incorrect or malicious inputs, improving user experience and data quality.
Handling Form Data
JavaScript allows dynamic interaction with form elements. Developers can access form fields using the DOM, retrieve values, and manipulate data before submission. Event listeners detect user actions like clicking submit buttons or changing input values.
To handle form data:
- Select the form element
- Add an event listener for form submission
- Prevent the default form action
- Gather form data using FormData or by accessing individual input values
- Process the data as needed (e.g. send to a server, update the page)
This approach gives developers full control over form behaviour and data management.
Implementing Client-side Validation
Client-side validation checks user input before form submission, providing immediate feedback and reducing server load. JavaScript can validate various input types, including text fields, emails, and passwords.
Common validation techniques:
- Check for empty fields
- Verify email format using regular expressions
- Validate password strength (length, character types)
- Compare password and confirmation fields
Validation functions can be triggered when a form is submitted or a user types a key. Error messages should be clear and specific, guiding users to correct their inputs.
Example validation code:
function validateEmail(email) {
const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return regex.test(email);
}
By implementing thorough client-side validation, developers can create more robust and user-friendly web forms.
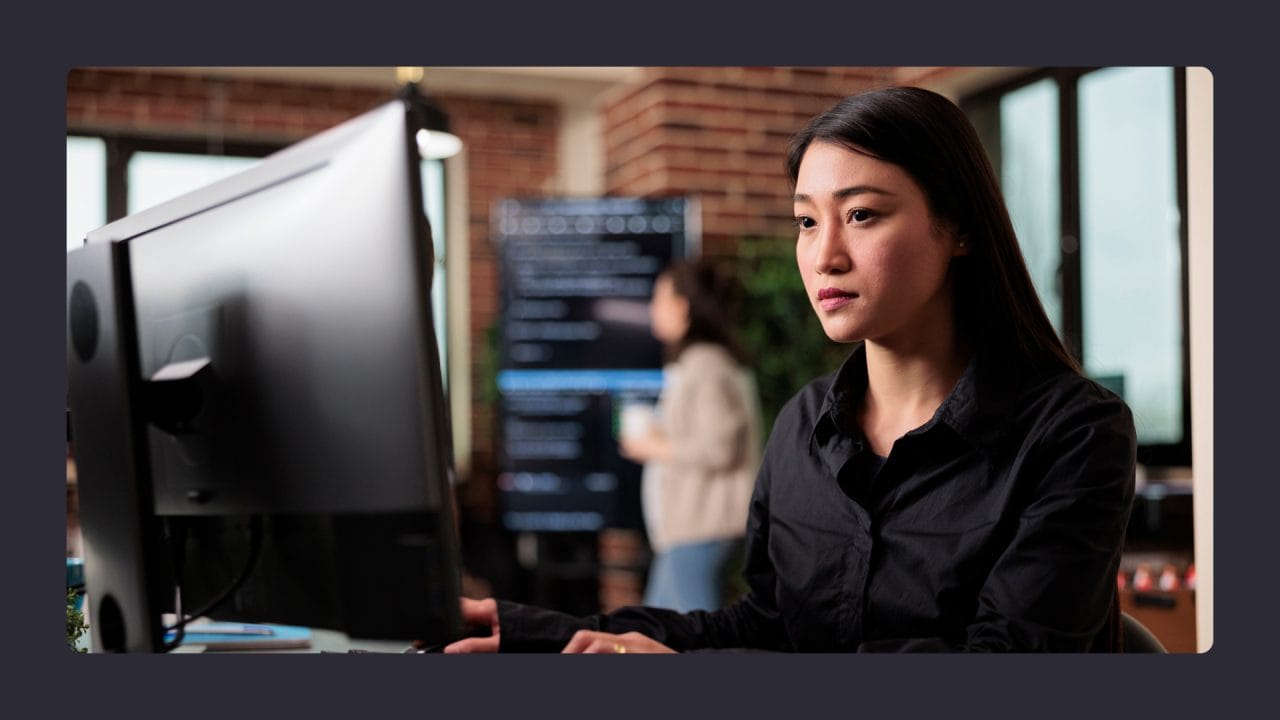
Incorporating External JavaScript Files
External JavaScript files separate code from HTML, improving organisation and reusability. This approach enhances maintainability and allows for easier updates across multiple web pages.
Organising Code with Modules
JavaScript modules help structure code into reusable, self-contained units. Each module can export functions, objects, or primitive values that others can import and use. This modular approach promotes cleaner code architecture and reduces the risk of naming conflicts.
To create a module, developers use the export
keyword to make certain parts of the code available to other files. The import
statement is then used to bring in these exported elements where needed. For example:
// math.js
export function add(a, b) {
return a + b;
}
// main.js
import { add } from './math.js';
console.log(add(2, 3)); // Outputs: 5
When incorporating external JavaScript files, the placement of the <script>
tag within the HTML file is essential. To ensure modules load correctly, include type="module"
in the <script>
tag, as follows:
<script type="module" src="main.js"></script>
This ensures that JavaScript modules are treated properly, enabling import and export functionality.
By carefully considering the loading and execution of JavaScript files, developers can optimise page performance and ensure a smooth user experience.
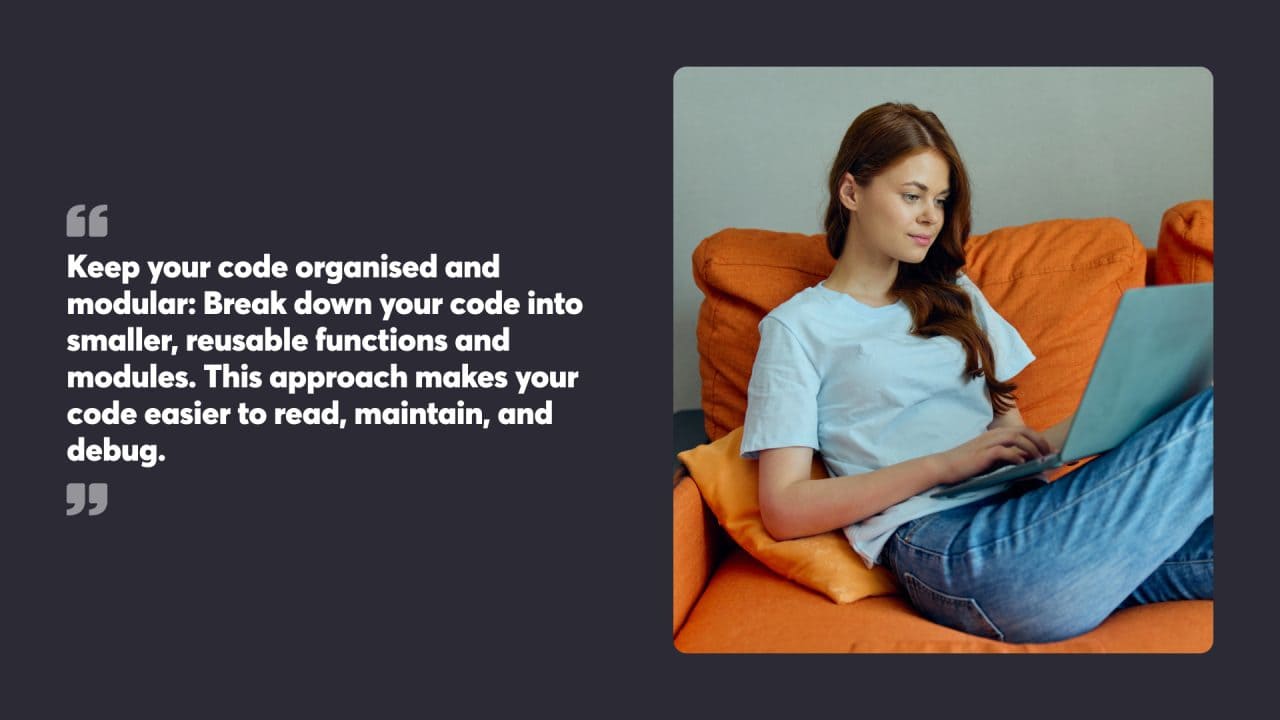
Best Practices for Writing JavaScript Code
Writing clean, maintainable, and efficient JavaScript code is essential for building dynamic and interactive web pages. Here are some best practices to keep in mind:
- Keep your code organised and modular: Break down your code into smaller, reusable functions and modules. This approach makes it easier to read, maintain, and debug.
- Use meaningful variable names and comments: Descriptive variable names and comments help explain what your code is doing, making it easier for others (and your future self) to understand and maintain.
- Use functions to encapsulate code: Functions allow you to modularise your code, enhancing readability and maintainability. Encapsulating code in functions also makes it easier to debug and reuse.
- Test your code thoroughly: Before deploying your code, test it thoroughly to ensure it works as expected and is error-free. This step is crucial for maintaining the reliability of your web pages.
- Use modern JavaScript syntax and features: Stay up-to-date with the latest JavaScript syntax and features, such as arrow functions, template literals, and destructuring assignments. These modern features can make your code more concise and easier to understand.
By following these best practices, you can write clean, maintainable, and efficient JavaScript code that enhances the interactivity and functionality of your web pages.
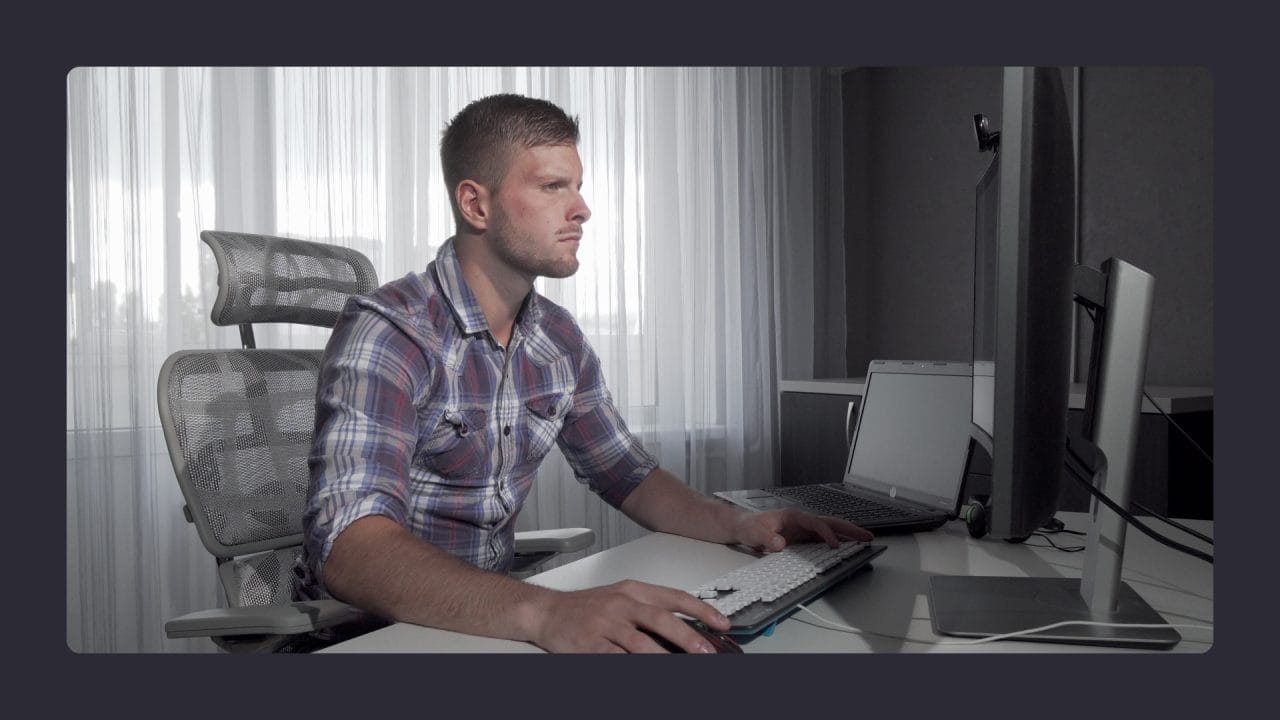
Real-World Applications of JavaScript
JavaScript has many real-world applications, from simple website animations to complex web applications. Here are some examples:
- Form validation: JavaScript can validate user input on forms, ensuring the data entered is correct and complete. This improves data quality and enhances the user experience.
- Interactive elements: JavaScript can create interactive elements such as sliders, forms, animations, and games, making websites more engaging and dynamic.
- Dynamic websites: JavaScript enables the creation of dynamic websites that adapt and respond to user interactions, providing a more personalised and responsive experience.
- Complex web applications: JavaScript is essential for building complex web applications, such as single-page applications (SPAs) and progressive web apps (PWAs). These applications offer a seamless user experience by loading content dynamically without refreshing the page.
- Mobile app development: JavaScript can be used to build apps using frameworks like React Native and Angular Mobile. These frameworks allow developers to create cross-platform mobile applications with a single codebase.
These examples highlight JavaScript’s versatility and power in web development. It is an indispensable tool for validating form data, creating interactive elements, or building complex web applications.
If it’s time to make your website more interactive, contact the team at Chillybin today.